13.Java中的GUI 【Java学习笔记Hatter】Java开发工程师
GUI
1.
Awt抽象窗口工具包,需要调用本地系统实现功能,属于重量级控件。
Swing在AWT基础上,完全由Java实现,属于轻量级控件。
2.布局管理器
(1)
FlowLayout流式布局管理器(从左到右,Panel默认的布局管理器)
BorderLayout边界布局管理器(东,南,西,北,中,Frame默认的布局管理器)
GridLayout网格布局管理器(规则的矩阵)
GardLayout卡片布局管理器(选项卡)
GridBagLayout网格包布局管理器(非规则的矩阵)
(2)
①创建frame窗体
②对窗体进行基本设置:比如大小,位置,布局。
③定义组件
④将组件通过窗体的add方法添加到窗体中
⑤让窗体显示,通过setVisible(true)
3.事件监听机制
特点:事件源、事件、监听器、事件处理
事件源:就是awt或swing包中的那些图形界面组件
事件:每一个事件源都有自己的特有的对应事件和共性事件
监听器:将可以触发某一个事件的动作都已经封装到了监听器中
以上三者,在Java中已定义,直接获取其对象来用就可以了
对产生的动作进行处理。
例:
public class FrameDemo {
public static void main(String[] args) {
Frame f = new Frame("my frame");
f.setBounds(400, 200, 500, 400);
f.setLayout(new FlowLayout());//设置流式布局
Button but = new Button("一个按钮");
f.add(but);//将按钮添加到窗体中。
f.addWindowListener(new WindowAdapter() {
public void windowClosing(WindowEvent e) {
//System.out.println("closing......."+e);
System.exit(0);
}
});
//在按钮上加上一个监听。
but.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
//System.out.println("button run .....");
System.exit(0);
}
});
f.setVisible(true);
System.out.println("over");
}
}
4.鼠标、键盘事件
例:
class MouseAndKeyDemo
{
private Frame f;
private Button b;
private TextField tf;
MouseAndKeyDemo(){
init();
}
public void init(){
f=new Frame("my frame");
f.setBounds(350,230,230,350);
f.setLayout(new FlowLayout());
tf=new TextField(20);
b=new Button("my button");
f.add(b);
f.add(tf);
myEvent();
f.setVisible(true);
}
private void myEvent(){
f.addWindowListener(new WindowAdapter(){
public void windowClosing(WindowEvent e){
System.exit(0);
}
});
tf.addKeyListener(new KeyAdapter() {
public void keyPressed(KeyEvent e){
int code=e.getKeyCode();
if(!(code>=KeyEvent.VK_0&&code<=KeyEvent.VK_9)){
System.out.println(code+"...是非法的");
e.consume();
}
}
} );
b.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
System.out.println("action ok");
}
});
b.addMouseListener(new MouseAdapter() {
private int count=1;
private int clickCount=1;
public void mouseEntered(MouseEvent e){
System.out.println("鼠标进入到该组件"+count++);
}
public void mouseClicked(MouseEvent e){
if(e.getClickCount()==2)
System.out.println("点击"+clickCount++);
}
});
b.addKeyListener(new KeyAdapter() {
public void keyPressed(KeyEvent e)
{
if(e.getKeyCode()==KeyEvent.VK_ESCAPE)//键盘按ESC键则终止程序并退出
System.exit(0);
System.out.println(KeyEvent.getKeyText(e.getKeyCode())+"..."+e.getKeyCode());
}
});
}
public static void main(String []args){
new MouseAndKeyDemo();
}
}
5.对话框
例:
public class MyWindowDemo {
private Frame f;
private TextField tf;
private Button b;
private TextArea ta;
private Dialog d;
private Label lb;
private Button ok_button;
MyWindowDemo() {
init();
}
public void init() {
f = new Frame("my window");
f.setBounds(300, 100, 600, 500);
f.setLayout(new FlowLayout());
tf = new TextField(60);
b = new Button("转到");
ta = new TextArea(25, 70);
d = new Dialog(f, "提示信息-self", true);
d.setBounds(400, 200, 240, 150);
d.setLayout(new FlowLayout());
lb = new Label();
ok_button = new Button("确定");
d.add(lb);
d.add(ok_button);
f.add(tf);
f.add(b);
f.add(ta);
myEvent();
f.setVisible(true);
}
private void myEvent() {
ok_button.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
d.setVisible(false);
}
});
tf.addKeyListener(new KeyAdapter() {
public void keyPressed(KeyEvent e)
{
if(e.getKeyCode()==KeyEvent.VK_ENTER)
showDir();
}
});
d.addWindowListener(new WindowAdapter() {
public void windowClosing(WindowEvent e) {
d.setVisible(false);
}
});
b.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
showDir();
}
});
f.addWindowListener(new WindowAdapter() {
public void windowClosing(WindowEvent e) {
System.exit(0);
}
});
}
private void showDir(){
String dirPath = tf.getText();
File dir = new File(dirPath);
if (dir.exists() && dir.isDirectory()) {
ta.setText("");
String[] names = dir.list();
for (String name : names) {
ta.append(name + "/r/n");
}
}
else {
String info = "您输入的信息:" + dirPath + "是错误的,请重新输入";
lb.setText(info);
d.setVisible(true);
}
tf.setText("");
}
public static void main(String[] args) {
new MyWindowDemo();
}
}
6.打开、保存文件
例:
public class MyMenuDemo {
private Frame f;
private MenuBar mb;
private Menu m,subMenu;
private MenuItem exit,son,open,save;
private TextArea ta;
private FileDialog openDia,saveDia;
private File file;
public MyMenuDemo() {
init();
}
public void init(){
f=new Frame("my frame");
f.setBounds(300,100,530,650);
mb=new MenuBar();
m=new Menu("菜单");
subMenu=new Menu("子菜单");
son=new MenuItem("子条目");
exit=new MenuItem("退出");
open=new MenuItem("打开");
save=new MenuItem("保存");
openDia=new FileDialog(f,"我要打开",FileDialog.LOAD);
saveDia=new FileDialog(f,"我要保存",FileDialog.SAVE);
ta=new TextArea();
subMenu.add(son);
m.add(subMenu);
mb.add(m);
m.add(open);
m.add(save);
m.add(exit);
f.setMenuBar(mb);
f.setVisible(true);
f.add(ta);
myEvent();
}
private void myEvent(){
save.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
if(file==null){
saveDia.setVisible(true);
String dirPath=saveDia.getDirectory();
String fileName=saveDia.getFile();
if(dirPath==null||fileName==null)
return;
file=new File(dirPath,fileName);
}
try {
BufferedWriter bw=new BufferedWriter(new FileWriter(file));
String text=ta.getText();
bw.write(text);
bw.flush();
bw.close();
} catch (IOException e1) {
throw new RuntimeException();
}
}
});
open.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
openDia.setVisible(true);
String dirPath=openDia.getDirectory();
String fileName=openDia.getFile();
System.out.println(dirPath+"..."+fileName);
if(dirPath==null||fileName==null)
return;
ta.setText("");
File file=new File(dirPath,fileName);
try {
BufferedReader br=new BufferedReader(new FileReader(file));
String line=null;
while((line=br.readLine())!=null){
ta.append(line+"/r/n");
}
} catch (FileNotFoundException e1) {
throw new RuntimeException("读取失败");
} catch (IOException e1) {
e1.printStackTrace();
}
}
});
exit.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
System.exit(0);
}
});
f.addWindowListener(new WindowAdapter() {
public void windowClosing(WindowEvent e) {
System.exit(0);
}
});
}
public static void main(String[] args) {
new MyMenuDemo();
}
}
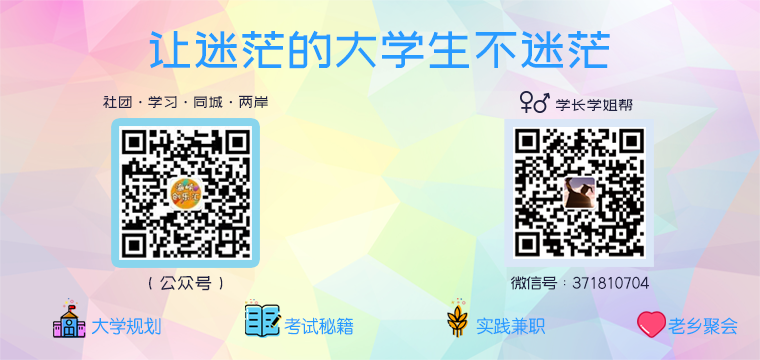
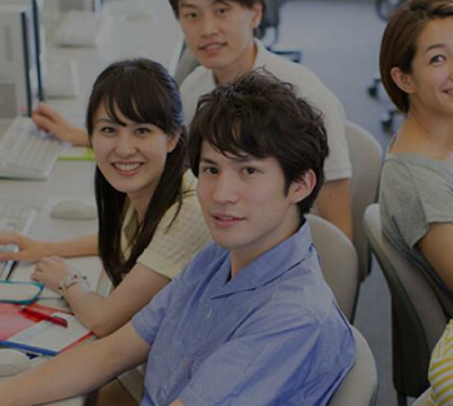
1914篇文章