2.Java中的函数与数组【Java学习笔记Hatter】Java开发工程师
循环结构
(1)while、do while与for
while:先判断条件,只有条件满足才执行循环体;
do while:先执行循环体,再判断条件,条件满足,再执行循环体,无论是否条件满足,循环体至少执行一次
for语句:
变量有其自己的作用域,对for来讲,若将用于控制循环的增量定义的for语句中,那么该变量只在for语句内有效,for完,该变量在内存中被释放。对于变量,若变量仅仅用于控制循环的次数作为循环增量存在用for。
(2)最简单无限循环
For(;;){} while(true){}
标号只用于循环
(3)break和continue语句
break:选择结构,循环结构。跳出循环
continue只能作用于循环结构。继续循环。特点:结束本次循环,继续下一循环
二、函数
1.函数就是方法
格式:
修饰符 返回值类型 函数名(参数类型 形式参数1,参数类型 形式参数2。。。){
执行语句;
return 返回值;}
特点:
(1)可将功能代码进行封装;
(2)提高了代码的复用性;
(3)当函数运算后,没有具体的返回值时,这是返回值类型用一个特殊关键字来表示void;
(4)函数中只能调用函数,不可以在函数内部定义函数。
如何定义一个函数?
(1)先明确该函数的运算结果,功能是什么?
(2)在明确定义功能的过程中是否需要未知的内容参与运算。
在同一个类中,允许存在一个以上的同名函数,只要他们的参数个数或者参数类型不同。
三、数组
1.数组
(1)数组即是一个容器,一次只能装同一类型数据。
(2)格式:元素类型[]数组名=new 元素类型[元素耳熟或数组长度]
int [] arr=new int[]{1,2,3,45,4};
int [] arr={1,2,3,4,5};
(3)栈内存:数据使用完毕,会自动释放(局部变量都在栈内存中开辟空间)
(4)堆内存:堆内存的每一个实体都有一个存放地址。存放实体,数组,对象(new)
ArrayIndexOutOfBoundsException:3:操作数组中不存在的角标 。
NullPointerException:空指针异常:当引用没有任何指向值为null的情况,该引用还在用于操作实体。
(5)length属性可以直接获取数组元素个数arr.length
(6)二维数组:二维数组本质上是以数组作为数组元素的数组,即“数组的数组”。
类型说明符 数组名[常量表达式][常量表达式]
2.数组的一些应用
(1)选择排序
public static void main(String[] args) {
int[] arr = new int[] { 3, 4, 2, 7, 33, 74, 753, 32 };
sop(arr);
selectSort(arr);
sop(arr);
}
public static void sop(int arr[]) {
System.out.print("[");
for (int i = 0; i < arr.length; i++)
if (i != arr.length - 1)
System.out.print(arr[i] + ",");
else
System.out.print(arr[i] + "]");
System.out.println();
}
public static void selectSort(int arr[]) {
for (int x = 0; x < arr.length - 1; x++) {
for (int y = x + 1; y < arr.length; y++) {
if (arr[x] > arr[y]) {
int temp = arr[y];
arr[y] = arr[x];
arr[x] = temp;
}
}
}
}
(2)冒泡排序(相邻的两个元素进行比较,若符合条件进行换位)
public static void main(String[] args) {
int[] arr = new int[] { 35, 4, 2, 3, 543, 6, 54 };
sop(arr);
bubbleSort(arr);
sop(arr);
}
public static void sop(int arr[]) {
System.out.print("[");
for (int i = 0; i < arr.length; i++)
if (i != arr.length - 1)
System.out.print(arr[i] + ",");
else
System.out.print(arr[i] + "]");
System.out.println();
}
public static void bubbleSort(int arr[]) {
for (int x = 0; x < arr.length - 1; x++) {
for (int y = 0; y < arr.length - x - 1; y++) {
if (arr[y] > arr[y + 1]) {
int temp = arr[y + 1];
arr[y + 1] = arr[y];
arr[y] = temp;
}
}
}
}
(3)折半查找(数组需有序)
public class ArrayHalfFind {
// 折半查找前提是有序的数组
public static void main(String[] args) {
int[] arr = new int[] { 1, 2, 5, 7, 9, 76 };
int index = halfFindMethod(arr, 2);
System.out.println(index);
}
public static int halfFindMethod(int arr[], int key) {
int min = 0, max = arr.length - 1, mid = (min + max) / 2;
while (arr[mid] != key) {
if (key > arr[mid])
min = mid + 1;
else if (key < arr[mid])
max = mid - 1;
mid = (max + min) / 2;
if (min > max)
return -1;
}
return mid;
}
}
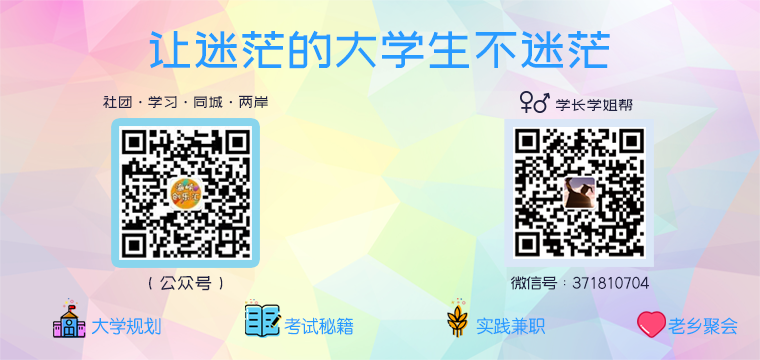
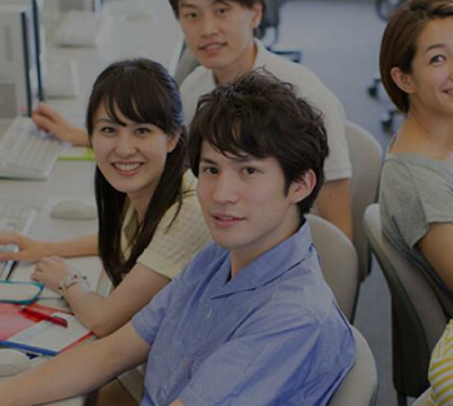
1914篇文章