15.Java中的正则表达式【Java学习笔记Hatter】Java开发工程师
符合一定规则的表达式
作用:用于专门操作字符串
特点:用已有一些特定的符号,来表示一些代码操作,这样简化书写
好处:可以简化对字符串的复杂操作。
弊端:符号定义越多正则越长,阅读性越差.
[abc]字符串当中某一个字符位出现的字符要么是a,要么是b,要么是c
JDK.LANG.STRING- matches-正则表达式
正则表达式常用操作:
1.匹配:String matches方法。用规则匹配整个字符串,只要有一处不符合,就匹配结束,返回false
2.切割:String split方法
(.)//1前一个任意字符,后一个与第一个相同一个或多个是+(叠词)
可以将规则封装成一个组,用()完成。
组的出现都有编号,从1开始,想要使用已有的组可以通过/n(n就是组的编号)的形式来获取
3.替换replaceAll
4.获取:将字符串中的 符合规则的子串去除
操作步骤:
①将正则表达式封装成对象
②让正则对象和要操作的字符串相关联
③关联后,获取正则匹配引擎
④通过引擎对符合规则的子串进行操作,比如取出。
group()用于获取匹配后的结果。
匹配
例1:
public class RegexDemo {
/*
* 求QQ号进行校验 要求:5~15 0不能开头,只能是数字
*/
public static void main(String[] args) {
checkQQ();
Demo();
}
public static void Demo(){
String str="f";
String regex="[a-z&&[def]]";
boolean b=str.matches(regex);
System.out.println(b);
}
public static void checkQQ(){
String QQ="2142sd342342342";
String regex="[1-9][0-9]{4,14}";//第一位1-9之间的数字,后面一位0-9之间的数字,数字范围4-14个之间
boolean flag=QQ.matches(regex);
if(flag)
System.out.println("OK"+flag);
else
System.out.println("NO"+flag);
}
}
例2:
/*
* 对邮件地址进行校验
*/
public class RegexTestDemo3 {
public static void main(String[] args) {
checkMail();
}
public static void checkMail(){
String mail="abc12@sina.com";
String reg="[a-zA-Z0-9_]+@[a-zA-Z0-9]+(//.[a-zA-Z]+)+";//较为精确的匹配
System.out.println(mail.matches(reg));
}
}
切割:
例3:
public class SplitDemo {
public static void main(String[] args) {
//spiltDemo("zhangsan.lisi.wangwu", "//.");
//spiltDemo("c://asdas//dasd", "////");
//spiltDemo("dsdaafsdaasdffs", "(.)//1");
//spiltDemo("dsdaaafsdddaasdfffs", "(.)//1+");
}
public static void spiltDemo(String str ,String reg){
String []arr=str.split(reg);
System.out.println(arr.length);
for(String s:arr){
System.out.println(s);
}
}
}
替换:
例4:
public class ReplaceAllDemo {
public static void main(String[] args) {
String str="dxfcgvhbjkml324563xcvhbjkml";//将字符串中的数字替换成#号
replaceAllDemo(str, "//d{5,}", "#");
String str1="jjsasdkksdawwdsad";//将叠词替换为#
replaceAllDemo(str1, "(.)//1", "&");
String str2="jjsasdkksdawwwwwwwwwdsad";
replaceAllDemo(str2, "(.)//1+", "&");
String str3="jjsasdkksdawwwwwwwwwdsad";
replaceAllDemo(str3, "(.)//1+", "$1");//规则中的第一个组
}
public static void replaceAllDemo(String str,String reg,String newStr){
str =str.replaceAll(reg, newStr);
System.out.println(str);
}
}
获取:
例:
public class GetRegexDemo {
public static void main(String[] args) {
getDemo();
}
public static void getDemo() {
String str = "ming tian jiu yao fang jia le da jia .";
String reg = "//b[a-z]{3}//b";
// 将规则封装成对象
Pattern p = Pattern.compile(reg);
// 让正则对象和要作用的字符串相关联
Matcher m = p.matcher(str);
/*
* String 类中的matches方法,用的就是Pattern和Matches对象来完成的
* 只不过被String的方法封装后,用起来比较为简单,但是功能却单一。
*/
while(m.find()){
System.out.println(m.group());
System.out.println(m.start()+"..."+m.end());
//boolean b = m.find();// 将规则作用到字符串上,并进行符合规则的子串查找
//System.out.println(b);
//System.out.println(m.group());
}
}
}
网络爬虫:
例:
public class RegexTest {
public static void main(String[] args) throws IOException {
getMails();
}
/*
* 获取指定文档中的邮件地址
* 使用获取功能Pattern Matcher
*/
public static void getMails1() throws Exception{
URL url=new URL("http://192.123.1.233:8080/myweb/mail.html");
URLConnection coon=url.openConnection();
BufferedReader brIn=new BufferedReader(new InputStreamReader(coon.getInputStream()));
String line=null;
String mailreg="//w+@//w+(//.//w+)+";
Pattern p=Pattern.compile(mailreg);
while((line=brIn.readLine())!=null){
Matcher m=p.matcher(line);
while(m.find()){
System.out.println(m.group());
}
}
}
public static void getMails() throws IOException{
BufferedReader br=
new BufferedReader(new FileReader("mail.txt"));
String line=null;
String mailreg="//w+@//w+(//.//w+)+";
Pattern p=Pattern.compile(mailreg);
while((line=br.readLine())!=null){
Matcher m=p.matcher(line);
while(m.find()){
System.out.println(m.group());
}
}
}
}
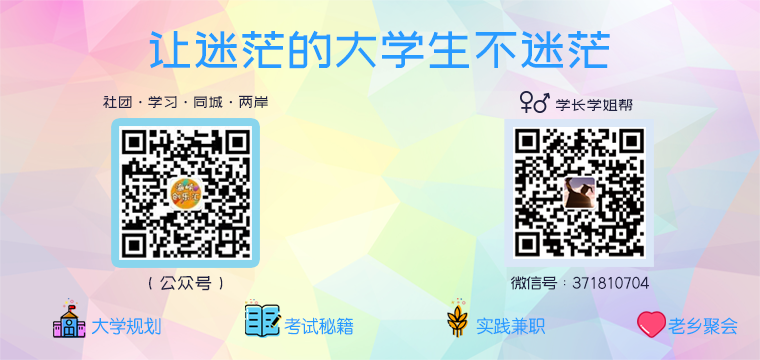
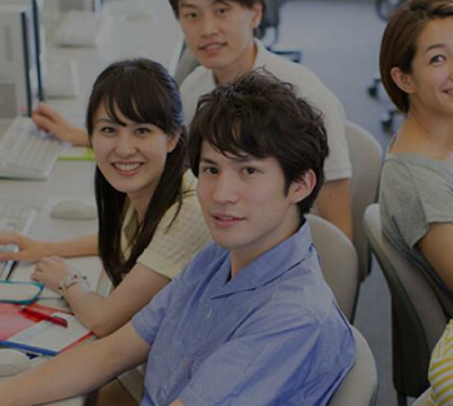
1914篇文章